2013
LiveLessons | informit.com
Paul Deitel
40+h
English
C# 2012 Fundamentals LiveLessons presents Paul Deitel's signature "Live Code" treatment of Microsoft's C# 2012 programming language - over 40 hours of expert video! Every important C# concept is presented in the context of a complete, working C# 2012 program. This LiveLesson contains over 20,000 lines of fully tested C# program code. And developers are free to reuse all of it.
Among the key topics included in this LiveLesson are:
-Windows 8 UI: Windows 8 UI style apps are called Windows Store apps. In Lesson 25, Deitel shows how to create and test Windows Store apps and upload them to Microsofts Windows Store.
-Databases and LINQ to Entities: The LINQ to SQL technology covered in the previous edition has been replaced with the more robust LINQ to Entities and the ADO.NET Entity Framework;
-Microsofts free SQL Server Express 2012 is used to present the fundamentals of database programming;
-ASP.NET 4.5, Microsofts .NET server-side technology, enables developers to create robust, scalable web-based apps;
-Windows Phone 8, Microsofts latest operating system for smartphones. It features multi-touch support for touchpads and touchscreen devices, enhanced security features and more. In Lesson 27 the author builds a complete working Windows Phone 8 app and test it in the Windows Phone simulator;
-Windows Azure, Microsoft's cloud computing platform that allows you to develop, manage and distribute apps in the cloud. Lesson 31 shows how to build a Windows Azure app;
-Asynchronous programming with async and await. Asynchronous programming is simplified in Visual C# 2012 with the new async and await capabilities.
Part I
C# 2012 Fundamentals LiveLessons Part I is an introduction to developing applications in the C# 2012 programming language. You begin by exploring the Visual Studio IDE. Then you learn about core C# constructs, including classes, objects, and methods. You move on to explore program control, and end with a lesson on exception handling.
Paul Deitel is co-founder of Deitel & Associates, Inc. and co-author of the best-selling C# 2012 For Programmers, 5/e. He is a Microsoft C# MVP. In this LiveLesson, Paul starts by showing viewers how to set up a C# development environment. He goes on to cover core C# programming concepts, including strings, classes, objects, control statements, and methods. Paul ends the lesson with an in-depth examination of arrays in C#.
Lesson 1: Setting Up Your Windows Environment
Introduction
Setting up your development environment
Lesson 2: Test-Driving a C# App Using Visual Studio for Windows Desktop
In this lesson, you learn to load and execute a project in Visual Studio Express 2012 for Windows Desktop
Introduction
Test-Driving a C# App Using Visual Studio for Windows Desktop
Lesson 3: Dive Into® Visual Studio Express 2012 for Windows Desktop
This lesson explores the Visual Studio Express 2012 for Windows Desktop Integrated Development Environment in more detail, including help features and key commands contained in the menus and toolbars.
Introduction
Overview of the IDE and creating a new project
IDE Menus and Toolbars
Navigating the IDE
Using Help
Using Visual App Development to Create a Simple App that Displays Text and an Image
Lesson 4: Introduction to C#
In this lesson you learn to write simple C# applications. Topics introduced include: classes,, namespaces, input/output statements, variables, operators and decision-making statements
Introduction
A Simple C# App: Displaying a Line of Text
Creating a Simple App in Visual Studio
Displaying a Single Line of Text with Multiple Statements
Displaying Multiple Lines of Text with a Single Statement
Formatting Text with Console.Write and Console.WriteLine
Adding Integers
Decision Making: Equality and Relational Operators
Lesson 5: Introduction to Classes, Objexts Methods and Strings
This lesson explores classes, objects, methods and instance variables. Topics covered include: class declarations, object creation, calling an object’s methods, and the use of constructors.
Introduction
Declaring a Class with a Method and Instantiating an Object of That Class
Declaring a Method with a Parameter
Instance Variables and Properties
Value Types vs. Reference Types
Initializing Objects with Constructors
Floating-Point Numbers, Type decimal and Validating Data
Lesson 6: Control Statements: Part 1
In this lesson you learn to use simple control statements, including if, if…else and while. The use of the prefix-increment, prefix-decrement, postfix-increment and postfix-decrement operators is also explored.
operators.
Introduction
Class Average Using Counter-Controlled Repetition
Class Average Using Sentinel-Controlled Repetition
Nested Control Statements
Compound Assignment Operators
Increment and Decrement Operators
Lesson 7: Control Statements: Part 2
This lesson explores more control statements, including the for, do/while, and switch statements. You learn to use both the end-of-file indicator to determine when user input is complete, and the boolean logical
Introduction
Essentials of Counter-Controlled Repetition
for Repetition Statement
Summing integers with the for statement
Compound Interest Calculations with for
do…while Repetition Statement
switch Multiple-Selection Statement
break Statement Exiting a for Statement
continue Statement Terminating an Iteration of a for Statement
Logical Operators
Lesson 8: Methods: A Deeper Look
In this lesson you learn how static methods and variables are associated with a class, random-number generation, what method overloading is, using recursion, and using methods with optional arguments.
Introduction
static Methods: User-Defined Method maximum
Three Ways to Call a Method
Promotion Rules
The .NET Framework Class Library
Shifted and Scaled Random Numbers for Rolling a Six-Sided Die
Roll a Six-Sided Die 6,000,000 Times
Case Study: A Game of Chance; Introducing Enumerations
Scope of Declarations
Method Overloading
Optional Parameters
Named Parameters
A Simple Example of Recursion: Factorial
Passing Arguments By Value and By Reference
Lesson 9: Arrays; Introduction to Exception Handling
This lesson explores arrays. Topics covered include how to: declare, initialize and refer to array elements, use the foreach statement to iterate through arrays, pass arrays to methods, and use command-line arguments.
Introduction
Creating an Array
Using an Array Initializer
Calculating a Value to Store in Each Element of an Array; Introducing const
Summing the Elements of an Array
Using Bar Charts to Display Data Graphically
Using the Elements of an Array as Counters
Using Arrays to Analyze Survey Results; Introduction to Exception Handling
Card Shuffling and Dealing Simulation; Introducing Arrays of References to Objects and the ToString method.
foreach Repetition Statement
Passing Arrays and Array Elements to Methods
Passing Arrays by Value and by Reference
Case Study: GradeBook Using an Array to Store Grades
Multidimensional Arrays
Case Study: GradeBook Using a Rectangular Array
Variable-Length Argument Lists
Using Command-Line Arguments
Part II
C# 2012 Fundamentals LiveLessons Part II picks up from where Part I left off. You begin by exploring Microsoft's LINQ database technology, then move on to a deeper dive into classes and objects. Next comes Paul Deitel's signature treatment of core OOP concepts: inheritance and polymorphism. The lesson ends with an exploration of Microsoft's GUI technology.
Paul Deitel is co-founder of Deitel & Associates, Inc. and co-author of the best-selling C# 2012 For Programmers, 5/e. He is a Microsoft C# MVP. In this LiveLesson, Paul picks up from where he left off in Part I. He begins with Microsoft's LINQ database technology, then proceeds to a deep treatment of C# classes and objects. Next comes the classic "Deitel treatment" of inheritance and polymorphism. Paul finishes the LiveLesson by covering exception handling and GUI programming with Windows Forms.
Lesson 10: Introduction to LINQ and the List Collection
This chapter explores basic LINQ concepts. Topics covered include how to: query an array using LINQ, create and use a generic List collection, and query a generic List collection using LINQ.
Introduction
Querying an Array of int Values using LINQ
Querying an Array of Employee Objects Using LINQ
Introduction to Collection and the Generic List Class
Querying a Generic Collection Using LINQ
Lesson 11: Classes and Objects: A Deeper Look
In this lesson you focus on encapsulation and data hiding. The "this" keyword is explored, as are the concepts of static data and methods, readonly fields, the Visual Studio Class View and Object Browser windows, and object initializers
Introduction
Time Class Case Study
Controlling Access to Members
Referring to the Current Object's Members with the this Reference
Time Class Case Study: Overloaded Constructors
Composition
static Class Members
A Note About Constant Fields of a Class
Class View and Object Browser
Object Initializers
Lesson 12: Object-Oriented Programming: Inheritance
This chapter explores how inheritance promotes software reusability. You learn how to create a derived class that inherits attributes and behaviors from a base class and how to reuse code.
Introduction
Base Classes and Derived Classes
Creating a CommissionEmployee Class
Creating a BasePlusCommissionEmployee Class without Using Inheritance
Creating a CommissionEmployee-BasePlusCommissionEmployee Inheritance Hierarchy
CommissionEmployee-BasePlusCommissionEmployee Inheritance Hierarchy Using protected Instance Variables
CommissionEmployee-BasePlusCommissionEmployee Inheritance Hierarchy Using private Instance Variables
Class object
Lesson 13: Object-Oriented Programming: Polymorpishm
Introduction
Demonstrating Polymorphic Behavior with the CommissionEmployee-BasePlusCommissionEmployee Hierarchy
Case Study: Payroll System Using Polymorphism
Creating Abstract Base Class Employee
Creating Concrete Derived Class SalariedEmployee
Creating Concrete Derived Class HourlyEmployee
Creating Concrete Derived Class CommissionEmployee
Creating Concrete Derived Class BasePlusCommissionEmployee
Polymorphic Processing, Operator is and Downcasting
Sealed Methods and Classes
Developing an IPayable Hierarchy
Declaring Interface IPayable
Creating Class Invoice
Modifying Class Employee to Implement Interface IPayable
Modifying Class SalariedEmployee for use with IPayable
Using Interface IPayable to Process Invoices and Employees Polymorphically
Operator Overloading
Lesson 14: Exception Handling: A Deeper Look
This lesson explores exceptions and how they are handled. You learn to use try and catch blocks, throw exceptions, use properties of class Exception, and create user-defined exceptions
Introduction
Example: Divide by Zero without Exception Handling
Example: Handling DivideByZeroExceptions and FormatExceptions
finally Block
The using Statement
Exception Properties
Programmer-Defined Exception Classes
Lesson 15: Graphical User Interfaces with Windows Forms: Part 1
In this lesson you learn to build simple Windows Forms GUIs. You learn to handle user-generated events, including mouse events and keyboard events. ToolTips and .Net namespaces are also explored.
Introduction
Introduction to GUIs
A Simple Event-Driven GUI
Building the Simple Event Example and Viewing the Auto-Generated GUI Code
Delegates and the Event Handling Mechanism
Locating Event Information
Control Properties and Layout
Labels, TextBoxes and Buttons
GroupBoxes and Panels
CheckBoxes
RadioButtons
PictureBoxes
ToolTips
NumericIUpDown Control
Mouse-Event Handling
Keyboard-Event Handling
Lesson 16: Graphical User Interfaces with Windows Forms: Part 2
This lesson continues the GUI discussion from lesson 12. You learn to build menuing systems and multiple document interface (MDI) programs, and you explore several common controls. You also learn to build custom controls.
Introduction
Menus
MonthCalendar Control
DateTimePicker Control
LinkLabel Control
ListBox Control
CheckedListBox Control
ComboBox Control TreeView Control
ListView Control
TabControl Control
Multiple Document Interface (MDI) Windows
Visual Inheritance
User-Defined Controls
Part III
C# 2012 Fundamentals LiveLessons Part III picks up where Part II leaves off. You begin by exploring string and characters in C#, then files and streams. Next comes Generics, Collections, anda deeper exploration of LINQ. The Lesson ends with a review of Microsoft's web application development technology and C# 2012's new asychronous programming features.
Paul Deitel is co-founder of Deitel & Associates, Inc. and co-author of the best-selling C# 2012 For Programmers, 5/e. He is a Microsoft C# MVP. In this LiveLesson, Paul picks up from where he left off in Part II. He begins with deep coverage of strings and characters, then moves on to files and streams and generics. Next comes collections, an expanded treatment of LINQ, and web app development in C#. Paul then skips to Lesson 26 to cover C#'s new asynchronous programming features.
Lesson 17: Strings and Chracters: A Deeper Look
This lesson takes a deep look at the string class and string objects. You learn to create mutable string objects using the StringBuilder class. The regular expression capabilities of the Regex and Match classes is also explored.
Introduction
string Constructors
string Indexer, Length property and CopyTo Method
Comparing strings
Determining Whether a String Begins or Ends with a Specified String
Locating Characters and Substrings in strings
Extracting Substrings from strings
Concatenating strings
Miscellaneous string Methods
Class StringBuilder Constructors
Length and Capacity Properties, EnsureCapacity Method and Indexer of Class StringBuilder
Append Method of Class StringBuilder
AppendFormat Method of Class StringBuilder
Insert and Remove Methods of Class StringBuilder
Replace Method of Class StringBuilder
Char Methods
Simple Regular Expressions and Class Regex
Regular-Expression Character Classes and Quantifiers
A More Complex Regular Expression
Validating User Input with Regular Expressions and LINQ
Regex Methods Replace and Split
Lesson 18: Files and Streams
This lesson explores file management. You learn to obtain information about files and directories, use LINQ to search through directories, and use classes FileStream and BinaryFormatter to read/write objects from/to files.
Introduction
Using Classes File and Directory
Using LINQ to Search Directories and Determine File Types
BankLibrary: Reusable Class BankUIForm
BankLibrary: Reusable Class Record
Creating and Writing to a Sequential-Access File
Reading Date from a Sequential-Access Text File
Case Study: Credit-Inquiry Program
BankLibrary: Class RecordSerializable
Creating a Sequential-Access File Using Object Serialization
Reading and Deserializing Data from a Binary File
Lesson 19: Generics
This lesson explores generic classes. You learn to manipulate data in a generic manner by learning how to implement your own generic types.
Introduction
Motivation for Generic Methods
Generic-Method Implementation
Type Constraints
Generic Classes
Generic Methods That Receive Objects of Generic Classes as Arguments
Lesson 20: Collections
This lesson explores collections. Both nongeneric and generic collections are presented. You explore the Array class and its methods, the use of enumerators to “walk through” a collection, and various important collection classes.
Introduction
Class Array and Enumerators
Class ArrayList
Class Stack
Class ClassHashtable
Generic Class SortedDictionary
Generic Class LinkedList
Lesson 21: Databases and LINQ
This lesson covers databases and LINQ. You learn to use LINQ to retrieve and manipulate data from a database. You explore the use of the ADO.NET Entity Data Model, the use of drag-and-drop techniques to quickly display database tables, and the use of data binding to move data seamlessly between GUI controls and databases.
Introduction
Relational Databases
Books Database
Books Database: Entity-Relationship Diagram
LINQ to Entities and the ADO.NET Entity Framework
Querying a Database with LINQ: Demonstrating the Display Authors Table App
Querying a Database with LINQ: Creating the ADO.NET Entity Data Model Class Library
Querying a Database with LINQ: Creating a Windows Forms Project and Configuring It to Use the Entity Data Model
Querying a Database with LINQ: Data Bindings Between Controls and the Entity Data Model
Querying a Database with LINQ: Code for the Data Bindings Between Controls and the Entity Data Model
Dynamically Binding Query Results
Dynamically Binding Query Results: Creating the Display Query Results GUI
Dynamically Binding Query Results: Coding the App
Retrieving Data from Multiple Tables with LINQ
Creating a Master/Detail View App
Address Book Case Study
Address Book Case Study: Creating the GUI
Address Book Case Study: Coding the app
Lesson 22: Web App Development with ASP.Net
This lesson covers Web application development with ASP.NET. You learn to handle events from a Web Form control, how to validate data on the client, maintain user-specific information with session tracking, and create data-driven applications.
Introduction
Web Basics
Multitier App Architecture
Testing Your First Web App
Building Your First Web App
Standard Web Controls
Validation Controls: Demo
Validation Controls
Session Tracking
Data Driven ASP.NET Guestbook: Demo
Data Driven ASP.NET Guestbook
Lesson 26: Asynchronous Programming with async and await
Introduction
Basics of async and await
Introduction to the Fibonacci calculation used in Sections 26.3-26.5
Executing an Asynchronous Task from a GUI App
Sequential Execution of Two Compute Intensive Tasks
Asynchronous Execution of Two Compute Intensive Tasks
Invoking a Flickr Web Service Asynchronously with WebClient
http://www.informit.com/store/c-sharp-2012-fundamentals-livelessons-parts-i-ii-iii-9780133443356
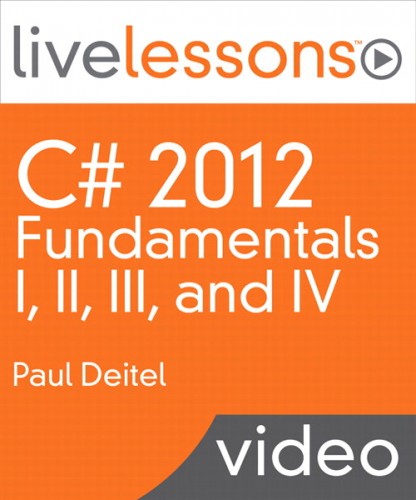
Download File Size:5.14 GB